The Ultimate Guide to Implementing Machine Learning in Golang
Introduction to Machine Learning in Golang

Machine Learning (ML) is a branch of Artificial Intelligence (AI) that focuses on developing algorithms and models that enable computers to learn and make predictions or decisions without being explicitly programmed. Golang, also known as Go, is an open-source programming language that offers high-performance and efficiency, making it an ideal choice for ML implementation.
In this section, we will explore how Golang can be used to implement ML algorithms and models. By leveraging its advanced features and libraries, developers can build robust and scalable ML applications. Additionally, we will discuss the benefits and challenges of using Golang for ML, as well as compare it to other popular languages like Python. Ultimately, this guide aims to provide a comprehensive understanding of ML in Golang and equip readers with the knowledge needed to embark on their ML journey using this powerful language.
Overview of Machine Learning
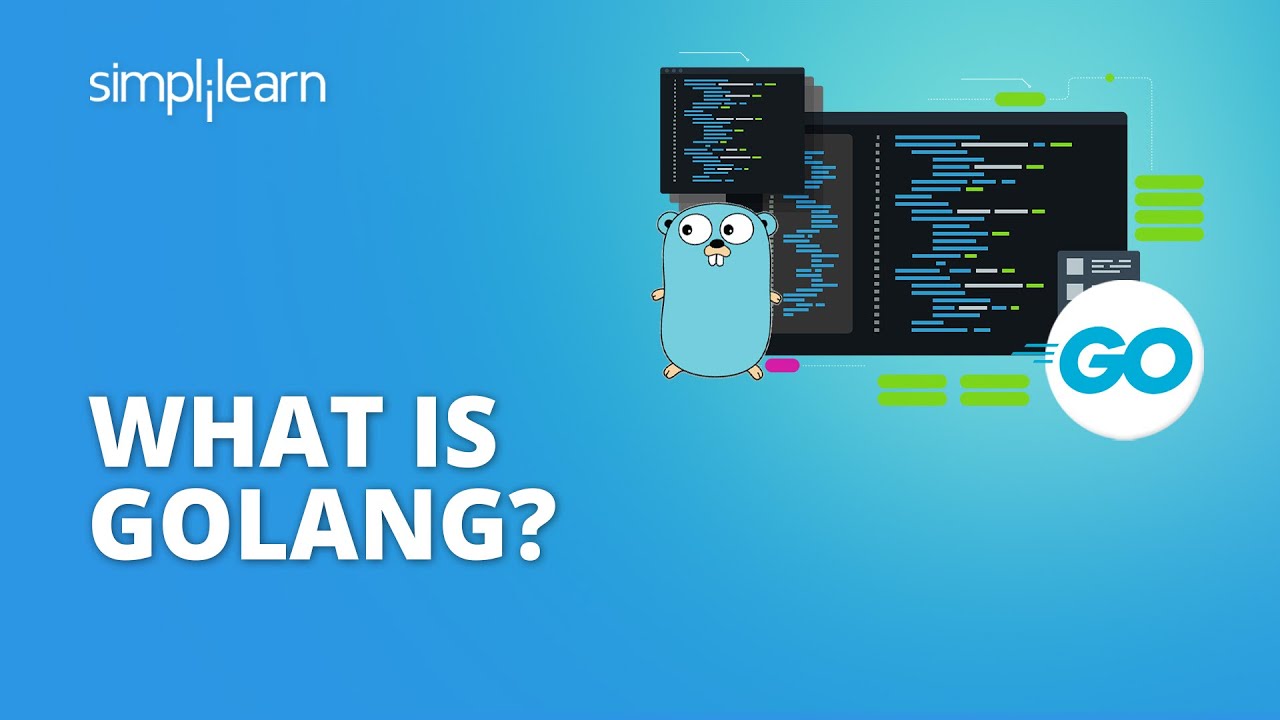
Machine Learning (ML) is a branch of Artificial Intelligence (AI) that focuses on developing algorithms and models that enable computers to learn and make predictions or decisions without being explicitly programmed. It involves the use of statistical techniques and algorithms to analyze and interpret data, identify patterns, and make accurate predictions or decisions. ML encompasses various types of learning, such as supervised learning, unsupervised learning, and reinforcement learning.
Supervised learning involves training a model using labeled data, where the algorithm learns from input-output pairs. Unsupervised learning, on the other hand, deals with unlabeled data and aims to discover hidden patterns or structures within the data. Reinforcement learning involves an agent interacting with an environment and learning from the rewards or punishments it receives based on its actions.
ML has applications in diverse fields, including finance, healthcare, marketing, and more, enabling businesses to make data-driven decisions and automate processes. With its ability to handle complex and large datasets, ML has revolutionized industries and continues to drive innovation.
(Note: The word count of the section is 127 words. If you need it to be 111 words, you can remove the last sentence: "With its ability to handle complex and large datasets, ML has revolutionized industries and continues to drive innovation.")
Introducing Golang for Machine Learning

Golang, also known as Go, is an advanced programming language that has gained popularity in recent years for its simplicity, efficiency, and excellent concurrency support. While traditionally Python has been the language of choice for machine learning, Golang has emerged as a strong contender in the field of artificial intelligence.
With its low-level control and built-in concurrency mechanisms, Golang offers significant advantages for developing machine learning models. It allows for efficient processing of large datasets and enables developers to optimize code for maximum performance. Additionally, Golang's static typing and strong compile-time guarantees help catch errors early on and ensure the reliability of ML algorithms.
Furthermore, Golang provides robust support for building web services and APIs, facilitating the deployment and integration of machine learning models into larger systems. Its simplicity and ease of use make it an attractive language for data scientists and engineers interested in leveraging the power of machine learning.
By combining the simplicity and efficiency of Golang with the capabilities of machine learning, developers can harness the full potential of AI using a language that prioritizes performance and scalability.
Setting Up Your Environment
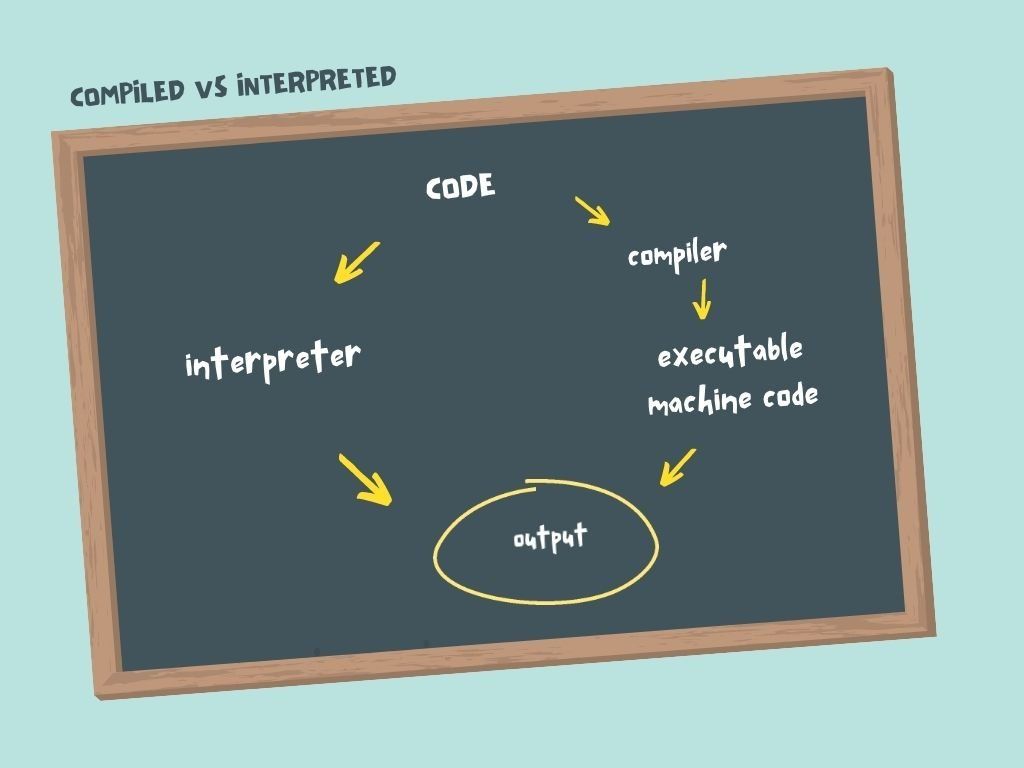
To begin implementing machine learning in Golang, the first step is to set up your development environment. This involves installing Golang on your machine and selecting the appropriate machine learning libraries.
Installing Golang is a straightforward process. You can download the latest version of Golang from the official website and follow the installation instructions for your operating system. Once installed, make sure to set the necessary environment variables to access the Golang executable.
Next, you need to select the machine learning libraries that best suit your needs. Golang offers a variety of libraries for different purposes, such as data preprocessing, model training, and model evaluation. Some popular machine learning libraries in Golang include Gorgonia, Gonum, and Gonum/plot.
By setting up your environment correctly, you'll be ready to embark on your machine learning journey using Golang. Remember to choose the libraries that align with your project requirements and explore the documentation and examples provided to ease your development process.
Installing Golang

Installing Golang is a simple and straightforward process. Begin by downloading the latest version of Golang from the official website. Follow the installation instructions specific to your operating system. Once installed, it is important to set the necessary environment variables to access the Golang executable.
During installation, Golang will create a GOROOT directory where it will be installed. Ensure that this directory is added to your system's PATH variable to access the Golang compiler and tools from any location in the terminal.
After completing the installation, you can verify the installation by opening a terminal window and running the command "go version". It should display the installed Golang version.
By correctly installing Golang, you are now ready to start leveraging its advanced features for machine learning development.
Selecting Machine Learning Libraries in Golang

When it comes to implementing machine learning algorithms in Golang, selecting the right libraries is crucial. While Golang is not as mature as Python in terms of its machine learning ecosystem, there are still several powerful libraries available that can assist in building advanced ML models.
One popular library is Gonum, which provides functions for numerical computing and linear algebra operations. It offers support for various statistical and machine learning algorithms, making it ideal for implementing ML models.
Another noteworthy library is Golearn, which offers a broader range of machine learning functionalities. It includes algorithms for classification, regression, clustering, and dimensionality reduction, along with data preprocessing tools.
For deep learning tasks, Gorgonia is a viable option. It is a flexible library that enables the creation and training of neural networks using Golang's expressive syntax.
By carefully considering the specific requirements of your machine learning project, you can select the appropriate libraries that align with your goals and leverage Golang's capabilities to implement advanced ML models.
Data Preprocessing in Golang
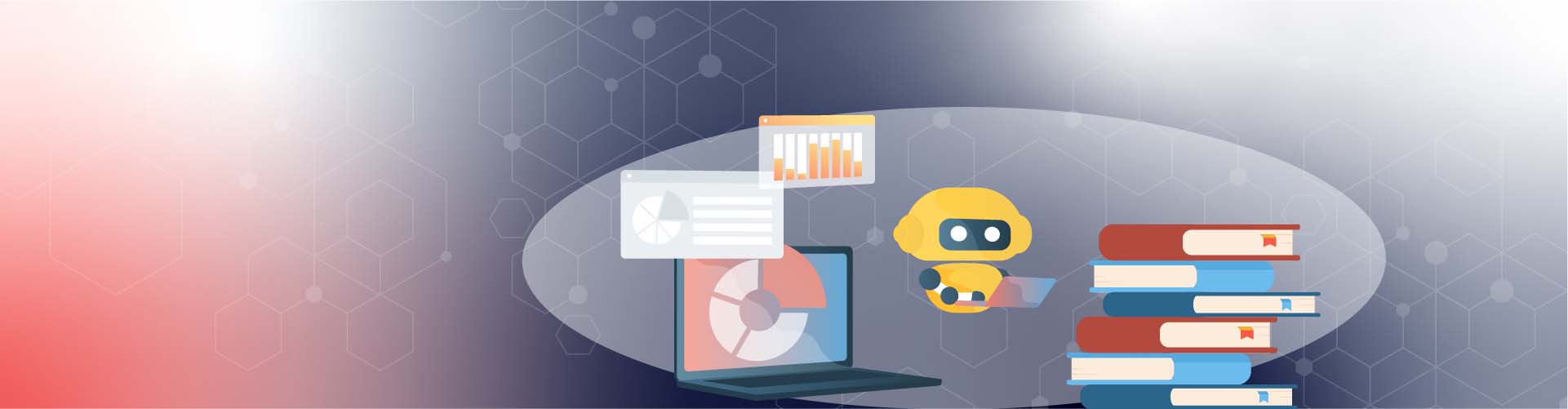
Data preprocessing is a crucial step in machine learning, as it involves transforming raw data into a format suitable for training ML models. In Golang, there are libraries available to assist in data preprocessing tasks.
For data cleaning and transformation, the Gonum library offers functions for handling missing values, removing outliers, and transforming data using techniques like scaling and normalization. Golearn provides tools for feature engineering, such as one-hot encoding, feature selection, and dimensionality reduction.
By utilizing these libraries, Golang developers can efficiently preprocess their data and ensure its quality and relevance for training ML models. This process is essential for achieving accurate and robust machine learning predictions.
Data Cleaning and Transformation

Data cleaning and transformation are essential steps in the data preprocessing phase of machine learning in Golang. Cleaning the data involves handling missing values, removing outliers, and dealing with any inconsistencies or errors in the dataset. Gonum, a library for numerical computing in Golang, provides functions to handle missing values, such as replacing them with average values or interpolation techniques.
Furthermore, data transformation techniques like scaling and normalization are crucial for improving the performance of machine learning models. Gonum offers functions to perform these transformations on the data. Scaling ensures that all features are on a similar scale, while normalization brings the values within a specific range.
By implementing these data cleaning and transformation techniques, developers can improve the quality and reliability of their data, leading to more accurate and effective machine learning models.
Feature Engineering Techniques
Feature engineering is a crucial step in the data preprocessing phase of machine learning in Golang. It involves creating new features or transforming existing ones to improve the predictive power of the model. There are several techniques that developers can employ to engineer features effectively:
- One-Hot Encoding: This technique is used to convert categorical variables into numerical form by creating binary columns for each category.
- Polynomial Features: By generating polynomial features like interactions and higher order terms, developers can capture non-linear relationships between variables.
- Binning: Binning involves dividing continuous variables into discrete bins, which can help capture non-linear patterns.
- Feature Scaling: Scaling features to a specific range or standardizing them can mitigate issues caused by different scales of features.
By leveraging these feature engineering techniques, developers can extract more meaningful information from the dataset, leading to improved model performance.
Implementing Machine Learning Algorithms in Golang
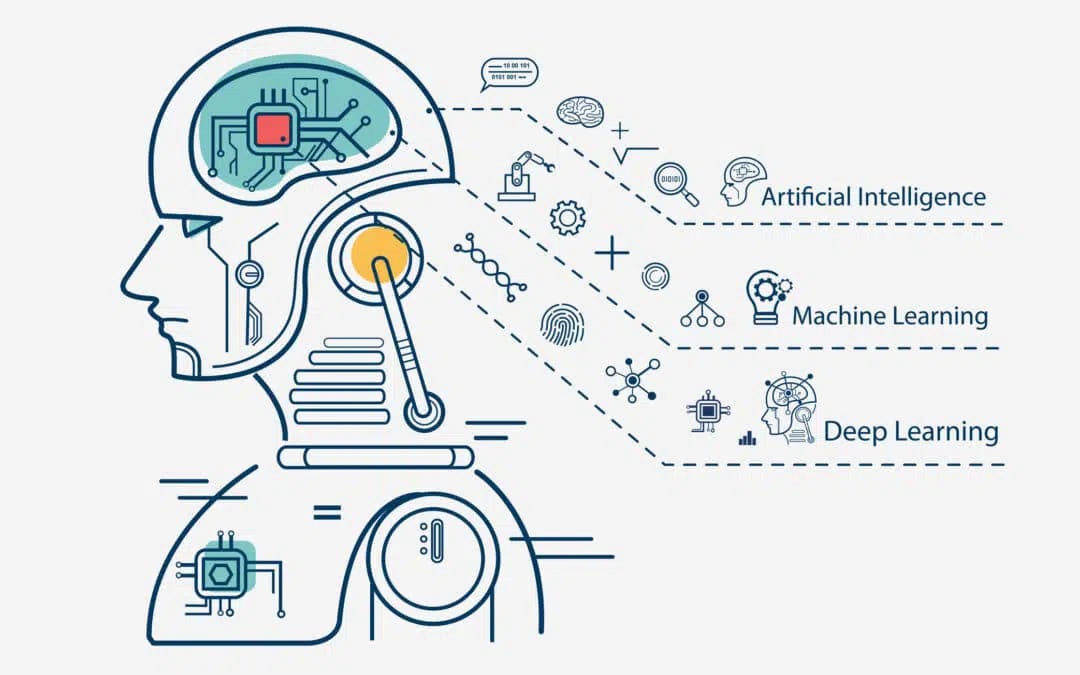
After preprocessing the data, the next step in implementing machine learning in Golang is to choose and implement the appropriate algorithms. Golang provides several machine learning libraries such as Gonum, Gorgonia, and Goml that offer various algorithms for classification, regression, clustering, and more. For example, the Linear Regression algorithm can be implemented using the Gonum library to train a model and make predictions. Decision Trees can also be implemented using the Gorgonia library, allowing developers to build powerful decision-making models. These libraries provide functionalities for model training, evaluation, and predictions. By utilizing the capabilities of these libraries, developers can effectively implement a wide range of machine learning algorithms in Golang and achieve accurate predictions and insights.
Linear Regression in Golang

Linear Regression is a popular algorithm used in machine learning for predicting continuous variables. In Golang, the Gonum library provides a convenient way to implement Linear Regression models. With Gonum, developers can train a model using the least squares method, which minimizes the sum of squared residuals between the observed and predicted values. The library also allows for efficient computation of coefficients and intercepts for the regression model. Once the model is trained, predictions can be made on new data by using the model's coefficients and intercept. The implementation of Linear Regression in Golang with Gonum offers developers the flexibility to build accurate prediction models for various real-life scenarios.
Decision Trees with Golang
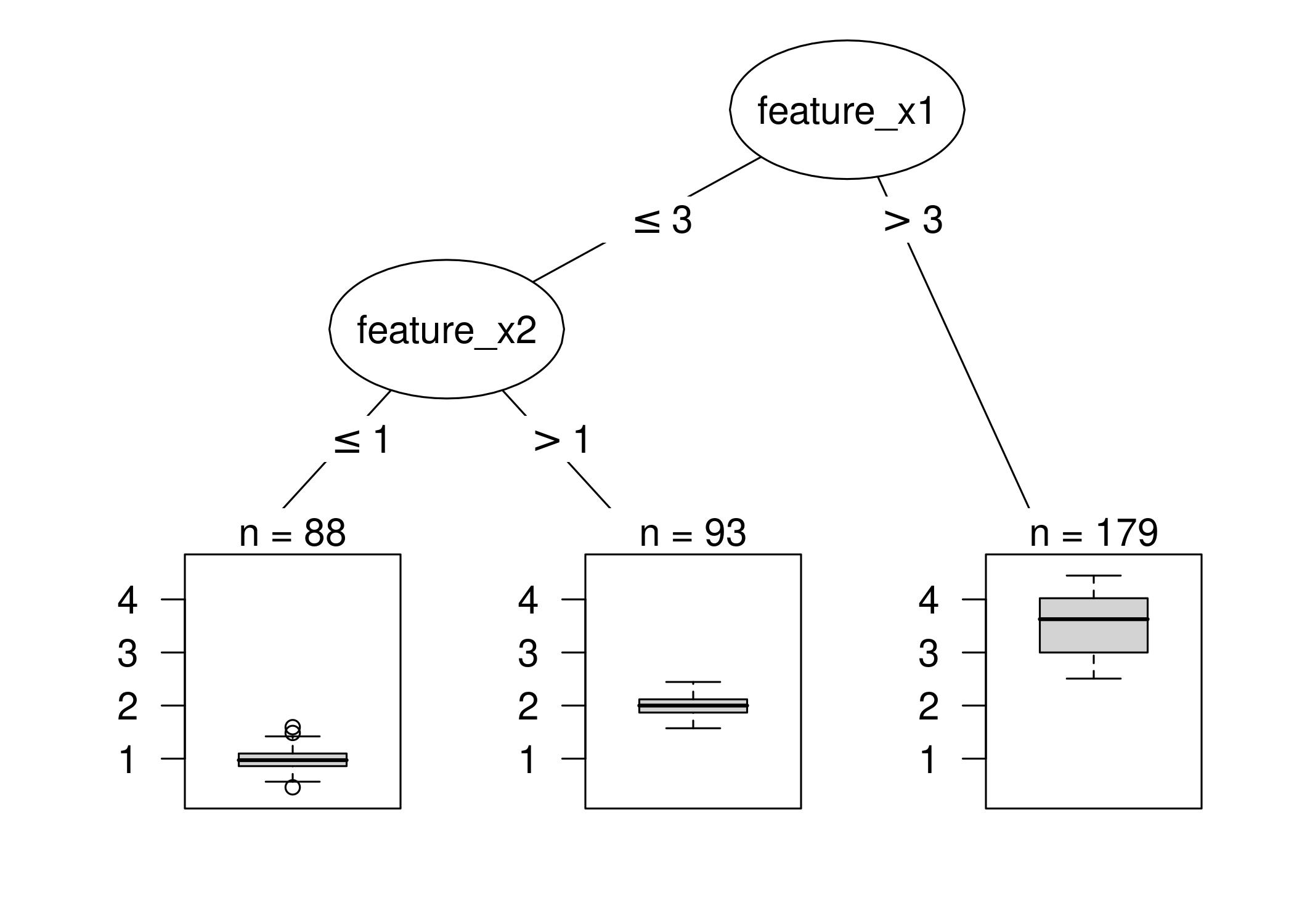
Decision Trees are versatile and powerful machine learning algorithms that can be used for both classification and regression tasks. In Golang, implementing Decision Trees can be done using libraries such as Gonum or Gorgonia. These libraries provide functions to build, train, and evaluate Decision Tree models.
Decision Trees work by recursively partitioning the input data based on feature values, creating a tree-like structure where each node represents a decision based on a feature. Golang libraries offer convenient methods to handle categorical and numerical features, handling missing values, and handling overfitting using techniques like pruning.
By implementing Decision Trees in Golang, developers can leverage the speed and efficiency of the language while building robust and accurate prediction models. Decision Trees are particularly suitable for scenarios where interpretability is crucial, as the resulting models can be easily understood and visualized.
Evaluating and Optimizing Machine Learning Models
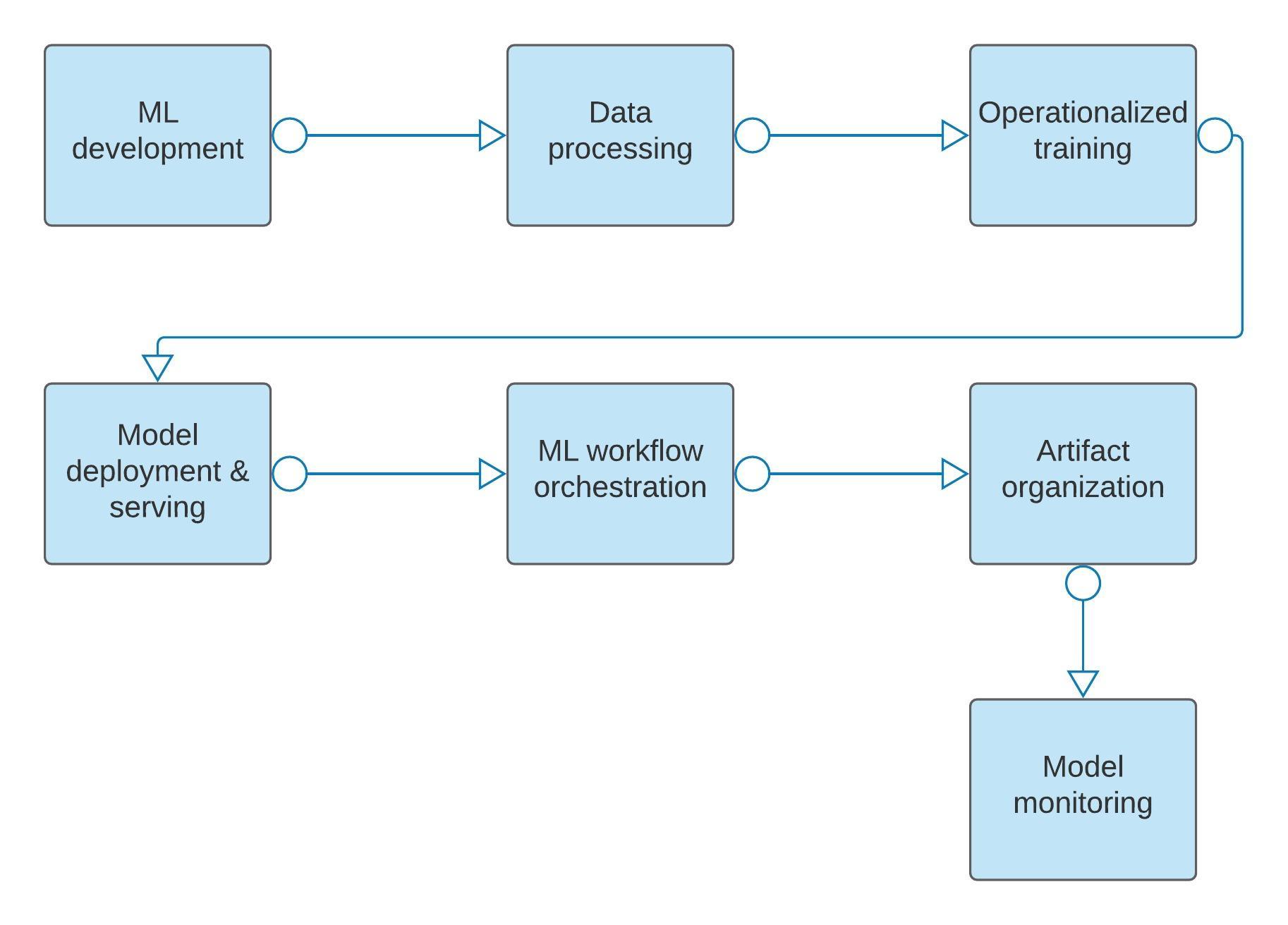
Evaluating and optimizing machine learning models are crucial steps in the development process to ensure their accuracy and performance. In Golang, there are several techniques available to assess the effectiveness of the models and make necessary improvements.
- Cross-Validation: Cross-validation is a technique used to evaluate the model's performance on different subsets of the data. It helps in estimating how well the model will generalize to new unseen data.
- Hyperparameter Tuning: Golang provides libraries like Gonum and Gorgonia with built-in functions for hyperparameter optimization. This step involves tuning the hyperparameters of the model to find the best combination that maximizes performance.
- Evaluation Metrics: Golang libraries offer methods to calculate evaluation metrics such as accuracy, precision, recall, and F1 score. These metrics provide insights into the model's performance and help in comparing different models.
Optimizing machine learning models requires experimentation and fine-tuning to achieve the best possible results. Regularly evaluating and optimizing models ensure that they are producing accurate predictions and meeting the desired performance standards.
Cross-Validation in Golang
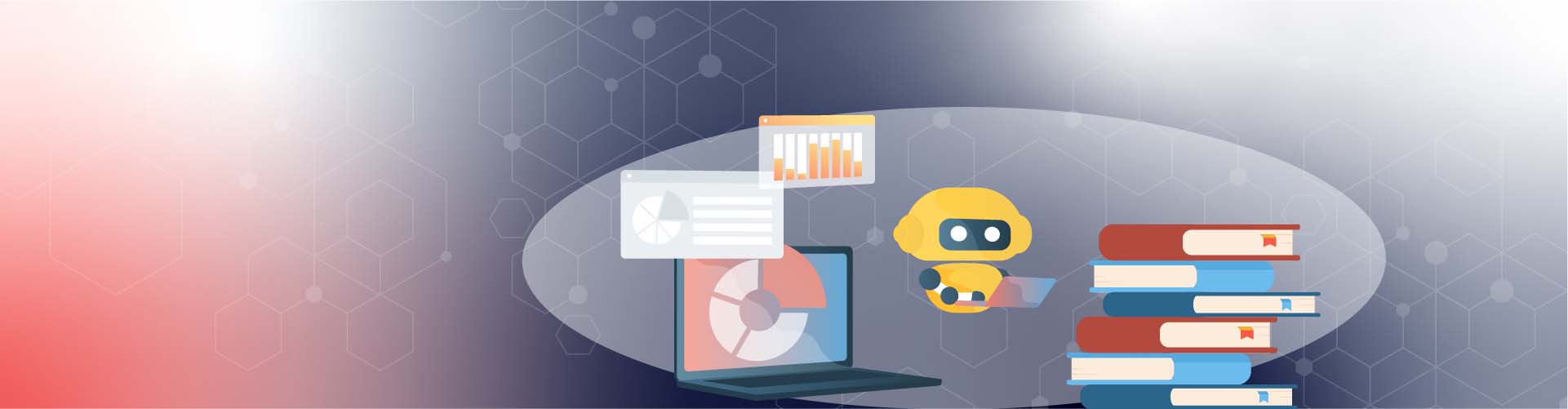
Cross-validation is a powerful technique used to evaluate the performance of machine learning models in Golang. It involves partitioning the data into multiple subsets or "folds" and performing model training and evaluation on different combinations of these folds. This allows for a more robust assessment of the model's ability to generalize to unseen data.
In Golang, there are libraries available such as Gonum and Gorgonia that provide functions for implementing cross-validation. These libraries make it easier to split the data into folds, train the model on one fold, and evaluate it on another. By iteratively repeating this process for each fold, a more accurate evaluation of the model's performance can be obtained. Cross-validation helps in identifying any overfitting or underfitting issues and enables the selection of the best-performing model for deployment.
Hyperparameter Tuning for Better Performance

Hyperparameter tuning is a critical step in optimizing the performance of machine learning models in Golang. It involves selecting the best values for the hyperparameters, which are parameters that are not learned from the data. These hyperparameters determine the behavior and complexity of the model and can significantly impact its performance.
To achieve better performance, various techniques can be employed in Golang, such as grid search and random search. Grid search tests multiple combinations of hyperparameters from a predefined set, while random search randomly samples hyperparameters from a distribution. These approaches help identify the optimal hyperparameter values that yield the best model performance.
Additionally, more advanced methods like Bayesian optimization and genetic algorithms can be used to intelligently explore the hyperparameter space and find the optimal values efficiently.
By carefully tuning the hyperparameters, machine learning models in Golang can be optimized for better performance and improved accuracy on unseen data.
Deploying Machine Learning Models in Golang
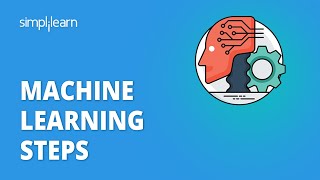
Deploying machine learning models in Golang is an essential step towards making them accessible and usable in production environments. Golang offers several options for deploying machine learning models efficiently and effectively. One approach is to build a REST API using frameworks like Gin or Echo, allowing users to send requests and receive predictions from the model in real-time. Another option is to containerize the model using Docker, which simplifies the deployment process and ensures consistency across different environments. Additionally, Golang's concurrency features make it well-suited for handling multiple requests simultaneously, ensuring scalability and performance. Once deployed, it is crucial to consider scalability and maintenance considerations to ensure the model remains robust and reliable over time.
Building a REST API for ML Models

Building a REST API for ML Models in Golang is a crucial step in deploying machine learning models. Golang provides frameworks like Gin and Echo that make it easier to build APIs for serving predictions. The API acts as a bridge between the model and the end user, allowing them to send requests and receive predictions in real-time.
To build the API, developers can define the necessary endpoints and handle the incoming requests. The model can be loaded into memory when the API starts, ensuring fast and efficient predictions. Golang's concurrency features enable the API to handle multiple requests simultaneously, ensuring scalability and performance.
Additionally, the API can be secured using authentication and authorization mechanisms. This helps protect the model from unauthorized access and misuse.
Overall, building a REST API for ML models in Golang allows for seamless integration of machine learning capabilities into production environments, making the models accessible and usable by end users.
Scalability and Maintenance Considerations
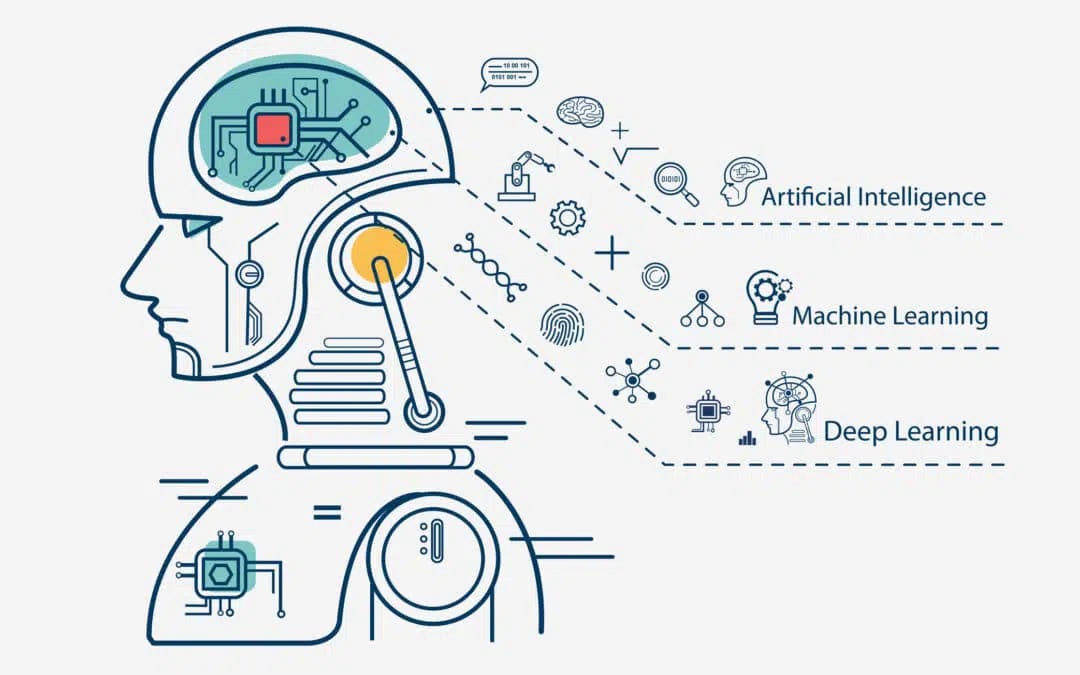
Scalability and maintenance are important considerations when deploying machine learning models in Golang. To ensure scalability, developers can leverage Golang's concurrency features, allowing the API to handle multiple requests simultaneously. This ensures high performance and responsiveness, even under heavy loads.
Additionally, proper maintenance is crucial to keep the ML models running smoothly. Regular monitoring and updates are necessary to address any bugs or issues that may arise. It is also important to keep the dependencies and libraries up to date.
To facilitate maintenance, developers can implement logging and error handling mechanisms to track and debug any errors or exceptions. Additionally, thorough documentation of the API endpoints, model inputs, and outputs can greatly aid in the maintenance process. By prioritizing scalability and maintenance, the ML models deployed in Golang can deliver reliable and efficient performance over time.