Machine Learning in Golang
Introduction to Machine Learning in Golang
Overview of machine learning and its application in Golang
Machine Learning (ML): A subset of artificial intelligence involving algorithms and data that enable machines to improve at tasks with experience.- **Application in Golang**: Golang, or Go, is gaining traction as a programming language for building scalable and high-performant ML models due to its simplicity and efficiency.- **Use Cases**: Go is often considered for system-level operations, but it is now becoming popular in areas such as data analysis, machine learning, and big data due to its performance benefits.Benefits of using Golang for machine learning projects
- **Performance**: Golang's static typing and compiled nature result in fast execution, which is beneficial for the heavy computations involved in ML.- **Concurrency**: Go's built-in concurrency model and goroutines make it easy to manage and process large datasets simultaneously, which is a typical requirement in ML tasks.- **Simplicity**: With a clean syntax, Golang simplifies the development process, making it easier for developers to write and maintain code for ML models.- **Community and Libraries**: Although Golang's ML ecosystem is not as rich as Python's, it has a growing community and a developing range of libraries and tools.- **Cross-Platform Development**: Golang's ability to compile to various platforms without changes to the codebase makes deploying ML models across different environments more straightforward.Essential Concepts in Machine Learning
Understanding supervised and unsupervised learning algorithms
- **Supervised Learning**: This involves mapping inputs to outputs based on example input-output pairs with the help of a supervisor or teacher. It's often used for classifications and regression tasks. - Examples include linear regression for predicting continuous outcomes and logistic regression for binary classification.- **Unsupervised Learning**: Contrary to supervised learning, unsupervised learning discovers hidden patterns or intrinsic structures in input data without labeled responses. - Clustering and dimensionality reduction are common unsupervised learning tasks, with k-means for clustering and principal component analysis (PCA) for feature reduction being widely recognized.Feature engineering and data preprocessing techniques
- **Data Preprocessing**: The process involves transforming raw data into an understandable format. It includes handling missing values, normalizing and scaling of data, and encoding categorical variables. - Data preprocessing is pivotal in enhancing the performance of machine learning models, ensuring that the data feeding into the models is clean and compatible.- **Feature Engineering**: This is the technique of extracting features from raw data that will improve the performance of learning algorithms. - It requires domain knowledge to create features that make the model more precise. Feature selection, feature extraction, and the creation of interaction features are part of this process.In the world of machine learning, grasping these foundational concepts is critical for anyone looking to leverage Golang's capabilities for developing efficient models, as they underpin the creation of algorithms capable of learning and adapting from data.
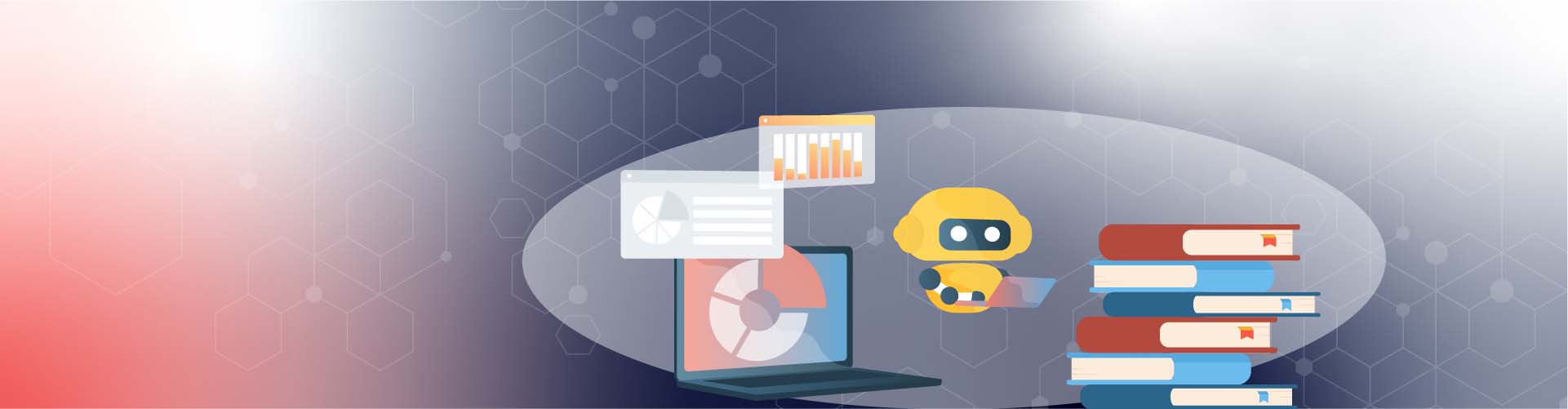
Golang Libraries for Machine Learning
Introduction to popular machine learning libraries in Golang
- GoLearn: It is a comprehensive machine learning library akin to scikit-learn in Python. Designed for ease of use and flexibility, GoLearn supports a wide range of machine learning tasks including classification, regression, and clustering.
- Gorgonia: This library is often likened to TensorFlow, providing the ability to define complex neural network graphs and perform automatic differentiation. Gorgonia is optimized for both performance and ease of use within the Go environment.
- Gonum: It offers a suite of numerical libraries for Go, including but not limited to statistics, linear algebra, and optimization. Gonum is instrumental for researchers engaged in scientific computing and applications that require advanced mathematical processing.
Comparison of Golang libraries for machine learning
Feature | GoLearn | Gorgonia | Gonum |
---|---|---|---|
Scope | Broad machine learning tasks | Neural networks and deep learning | Scientific computing |
Ease of Use | High (similar to scikit-learn) | Moderate (more complex for deep learning) | Moderate to High (depends on task complexity) |
Flexibility | Moderate | High (can define complex models) | High (versatile numerical capabilities) |
Community Support | Moderate | Growing | Strong in scientific community |
Performance | Good | Very High | Excellent for numerical tasks |
In the professional realm, comprehending these libraries' capabilities and distinctions is valuable for developers and data scientists seeking efficient and tailored solutions in Golang. They offer a range of functionalities from basic algorithms to complex neural network architectures, thus enabling diverse machine learning applications to be built within the robust and performant Go ecosystem.
Supervised Learning Algorithms in Golang
Linear regression and logistic regression in Golang
Professionals leveraging Golang for data-driven applications have at their disposal powerful libraries for supervised learning. **GoLearn**, for example, offers implementations of both linear regression and logistic regression. These algorithms are fundamental to predictive analytics, where linear regression is used for forecasting continuous outputs and logistic regression for binary classification tasks. Through GoLearn, the process of training and deploying these models is streamlined, thereby allowing practitioners to focus more on model tuning and interpretation of results.Decision trees and random forests in Golang
For data professionals seeking to implement decision tree algorithms, GoLearn provides a reliable framework within the Golang ecosystem. On top of that, Golang enthusiasts can leverage ensemble methods like random forests offered by the same library. Decision trees excel in handling non-linear datasets with complex patterns, while random forests aggregate multiple trees to boost prediction accuracy and control over-fitting. The integration of these algorithms into Go applications capitalizes on the concurrency features of Go, facilitating efficient model training and prediction on large-scale datasets.Utilizing these tools, professionals can build predictive models that not only perform well but also scale efficiently with Go's robust system-level capabilities.

Unsupervised Learning Algorithms in Golang
K-means clustering and hierarchical clustering in Golang
Within the Golang community, data scientists have adopted robust libraries to implement unsupervised learning algorithms. For cluster analysis, **GoLearn** provides a cohesive API for K-means clustering, which partitions data into k distinct clusters based on features. Furthermore, hierarchical clustering is accessible through the same package, enabling analysts to create a tree of clusters. These methodologies are essential for exploratory data analysis, where determining the intrinsic grouping in unlabeled datasets is crucial.Principal Component Analysis (PCA) in Golang
Additionally, Go users focusing on dimensionality reduction can utilize Principal Component Analysis (PCA) functionalities available in Golang libraries like Gonum. PCA is a sophisticated statistical technique that transforms a set of observations of possibly correlated variables into a set of values of linearly uncorrelated variables. This is particularly beneficial when dealing with high-dimensional data, allowing for simplification of the dataset while retaining most of the original variability.By integrating these advanced algorithms, Golang practitioners are equipped to explore complex datasets and uncover hidden structures, all while leveraging Go's exceptional performance and concurrency capabilities. These undertakings enhance the potential to derive actionable insights from large volumes of data with increased efficiency and accuracy.

Evaluating Machine Learning Models in Golang
Cross-validation and performance metrics in Golang
In the realm of machine learning within the Go environment, meticulous evaluation of models is imperative. Golang developers often employ cross-validation techniques to ascertain the efficacy of their unsupervised learning models. This process involves partitioning the data set and iteratively training and validating the model on distinct sets, which ensures that the model's performance is consistent across different subsets of data.
They also rely on a variety of performance metrics to measure the precision and robustness of these models. For instance, the Silhouette Coefficient is commonly used to assess the quality of clustering in K-means, while metrics like mutual information score provide insights into the shared information between hierarchical clustering outputs and the true labels, should they be available.
Overfitting and underfitting in machine learning models
Concerning model reliability, practitioners are vigilant about the pitfalls of overfitting and underfitting. Overfitting occurs when a model learns the noise and random fluctuations in the training data to the extent that it negatively impacts the model's performance on new, unseen data. In contrast, underfitting refers to a model that is too simple to capture the underlying pattern in the data, thereby performing poorly even on the training dataset. To combat these issues, professionals might use techniques such as Pruning in hierarchical clustering, or adjusting the number of clusters in K-means to optimize the trade-off between model complexity and generalization performance.
The Go community continues to enrich its portfolio of machine learning tools, thereby empowering developers to perform comprehensive model evaluations with precision and to ensure their models possess the requisite generalizability for real-world applications.
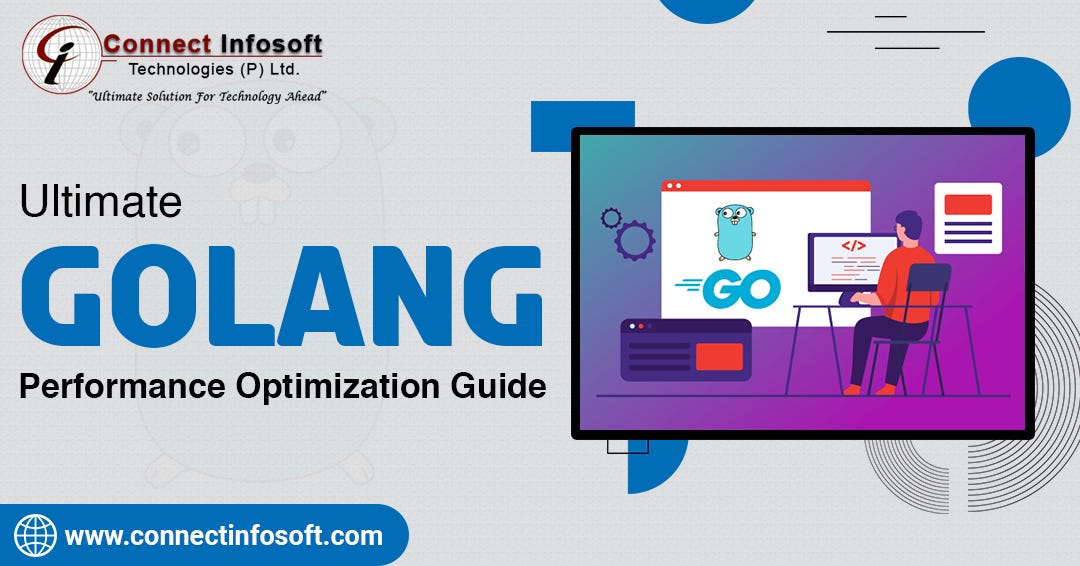
Feature Selection and Dimensionality Reduction in Golang
Techniques for selecting relevant features in Golang
In Go's machine learning development sphere, feature selection stands as a pivotal step to enhance model performance. By meticulously choosing only the most informative and relevant features, developers can significantly reduce model complexity without sacrificing efficacy. Techniques such as Recursive Feature Elimination (RFE) and feature importance ranking emerged as favored methodologies within the Golang community. Notably, RFE iteratively constructs models and removes the weakest feature at each iteration, refining the model's predictive capacity. Concurrently, feature importance ranking, often derived from model coefficients or tree-based methodologies, guides developers in discerning which features contribute most significantly to the predictive modeling process.
Dimensionality reduction methods in Golang
Go aficionados leverage various dimensionality reduction methods to strip down data to its most essential form. Techniques such as Principal Component Analysis (PCA) and t-Distributed Stochastic Neighbor Embedding (t-SNE) are integral in this process. PCA, through orthogonal transformation, reduces the dataset to a set of linearly uncorrelated principal components, henceforth consolidating data interpretability. Alternatively, t-SNE offers a probabilistic approach to dimensionality reduction, adept at preserving the local structure of high-dimensional data, making it invaluable for visual cluster identification in complex datasets. These reduction methods offer a balance between preserving significant information and simplifying the dataset, thus enabling more efficient computation and potentially improved model performance.

Deploying and Scaling Machine Learning Models in Golang
Integration of machine learning models into production systems
In the realm of machine learning development using Golang, the deployment and integration of models into production systems is a critical step. It involves seamlessly incorporating trained models into existing software infrastructure for real-time predictions. With Golang's strong support for concurrency and efficient memory management, integration becomes relatively straightforward. Developers can leverage frameworks like TensorFlow or GoCV to deploy machine learning models within Golang applications. Additionally, using libraries such as gRPC or RESTful APIs, models can be seamlessly exposed as web services, allowing other applications to easily consume the predictions.
Scalability considerations for machine learning projects in Golang
When it comes to scaling machine learning projects in Golang, developers must consider various factors to ensure optimal performance. Golang's lightweight goroutines and efficient memory management make it well-suited for handling concurrent tasks and scaling resources. Utilizing load balancers and distributed systems architectures, such as Kubernetes or Docker Swarm, can help distribute the computational workload across multiple machines, allowing machine learning models to handle large-scale data efficiently. Additionally, implementing caching mechanisms can further improve scalability by reducing the load on the machine learning models.
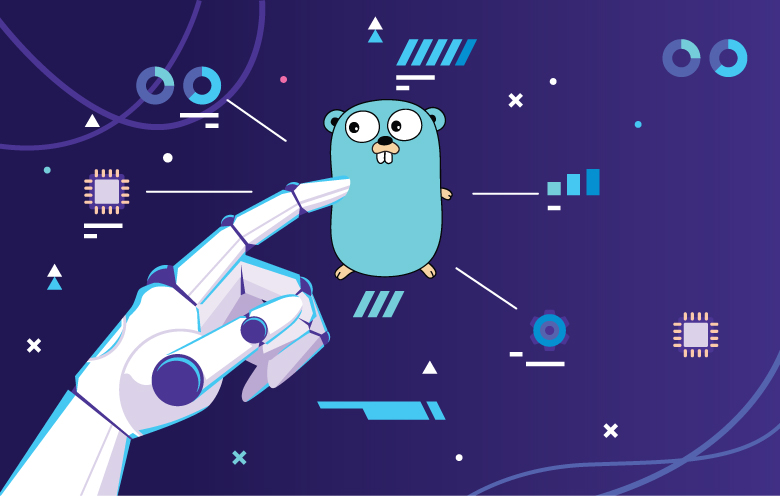
Conclusion
Summary of machine learning in Golang
In summary, when deploying and scaling machine learning models in Golang, developers can leverage the language's support for concurrency and efficient memory management to seamlessly integrate trained models into production systems. Frameworks like TensorFlow and GoCV can be used to deploy models within Golang applications, while libraries such as gRPC or RESTful APIs can expose models as web services for easy consumption by other applications.
When it comes to scalability, Golang's lightweight goroutines and efficient memory management make it well-suited for handling concurrent tasks and scaling resources. Load balancers and distributed systems architectures, like Kubernetes or Docker Swarm, can distribute the computational workload across multiple machines, enabling efficient handling of large-scale data. Implementing caching mechanisms can further improve scalability by reducing the load on the machine learning models.
Future trends and advancements in the field
Looking ahead, the field of machine learning in Golang is expected to see further advancements and trends. This includes the development of more specialized libraries and frameworks, specifically tailored for machine learning tasks in Golang. Additionally, advancements in hardware and infrastructure technologies, such as GPUs and cloud computing, will continue to enhance the performance and scalability of machine learning projects.Furthermore, the integration of automated machine learning (AutoML) capabilities into Golang frameworks and libraries will simplify the model deployment and scaling process even further. This will allow developers to streamline the end-to-end machine learning development workflow, from data preparation and model training to deployment and scaling.
Overall, deploying and scaling machine learning models in Golang offers developers a powerful and efficient solution. With its support for concurrency and scalability, Golang enables seamless integration of machine learning models into production systems, paving the way for the development of intelligent applications across various industries.